事实上,【勤之时】一个本地应用,基本上没有什么网络请求,或者需要从服务器端下载数据。不过为了美观,每天的背景图片会变化,并且会有一个每日故事的分享页面,也就是说,每天一图。
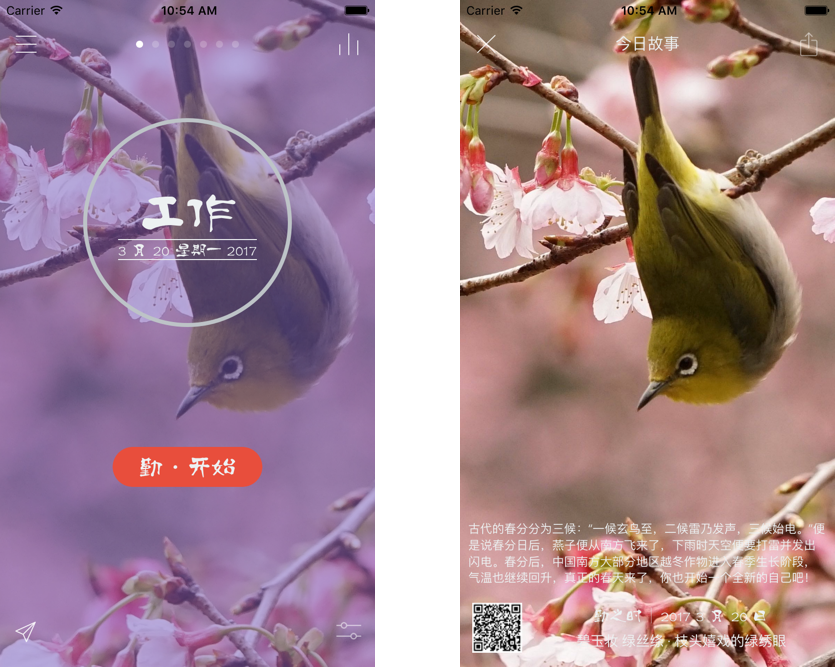
那么具体这个图片怎么来?当然可以自己搭建服务器,然后提供图片。不过这样相对来说比较复杂,不但要搭建服务器,还要自己准备图片。所以最简单的是看有没有现成的API 服务,我找到了两个:
Bing的每日一图并不是API服务,不过通过抓包,可以发现http://www.bing.com/HPImageArchive.aspx?format=js&idx=0&n=1 可以获取到无水印的图片。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
{
"images": [{
"startdate":"20170319",
"fullstartdate":"201703191600",
"enddate":"20170320",
"url":"/az/hprichbg/rb/TingSakura_ZH-CN14945610051_1920x1080.jpg",
"urlbase":"/az/hprichbg/rb/TingSakura_ZH-CN14945610051",
"copyright":"一只在樱花树上嬉戏的绿绣眼(© Reece Cheng/500px)",
"copyrightlink":"http://www.bing.com/search?q=%E6%98%A5%E5%88%86&form=hpcapt&mkt=zh-cn",
"quiz":"/search?q=Bing+homepage+quiz&filters=WQOskey:%22HPQuiz_20170319_TingSakura%22&FORM=HPQUIZ",
"wp":true,
"hsh":"c4afb61170efde716ed371c02f8db13f","drk":1,"top":1,"bot":1,"hs":[]
}],
"tooltips": {
"loading":"正在加载...",
"previous":"上一个图像",
"next":"下一个图像",
"walle":"此图片不能下载用作壁纸。",
"walls":"下载今日美图。仅限用作桌面壁纸。"
}
}
从返回的Json中可以看到,我们可以获取到urlbase,经过测试,bing提供了1080x1920的图片,其后缀为_1080x1920.jpg,所以最后无水印图片的下载地址为http://www.bing.com/urlbase_1080x1920.jpg。
那么当前图片对应的故事在哪里呢?同样发现了API接口http://cn.bing.com/cnhp/coverstory/,不过这里的图片是有水印的。
1 | { |
所以综合这两个,我们就可以得到每日背景图片及故事。
至于Unsplash,大家可以参考Unsplash官网的说明去试试。【勤之时】暂时使用的是Bing的每日一图及故事来作为背景。
知道了需求,了解了内容的来源,接下来就是如何实现了。同样,iOS应用架构谈 网络层设计方案探讨了详细的方案。
最终的方案
- 使用delegate来做数据对接
- 交付NSDictionary给业务层,使用Const字符串作为Key来保持可读性
此外,【勤之时】的需求涉及到同步问题,可以发现,我们的操作为
- 访问http://www.bing.com/HPImageArchive.aspx?format=js&idx=0&n=1 获取Json数据
- 根据Json数据拼接获得每日图片的下载地址
- 下载图片
- 访问http://cn.bing.com/cnhp/coverstory/获取每日故事的内容。
可以发现,我们会发送3个HTTP异步请求,只有当三个请求全部成功返回后,我们才能认为这个下载任务完成。因此,需要使用dispatch_group_t, dispatch_group_enter, dispatch_group_leave,dispatch_group_notify来完成这个组合。
最终代码:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18// ILDNetwork.h
#import <Foundation/Foundation.h>
@protocol ILDNetworkDelegate <NSObject>
- (void)fetchStoryDataSuccess:(NSDictionary *)storyDataDictionary;
- (void)fetchStoryDataFail:(NSError * _Nonnull)error;
@end
@interface ILDNetwork : NSObject
@property (nonatomic,weak) id<ILDNetworkDelegate> delegate;
- (void)downloadStoryData;
@end
1 |
|